Development of AI Chatbot for Telegram with Python and MySQL
Learn how to develop an AI-powered Telegram chatbot using Python and MySQL with a step-by-step guide. Enhance automation, user experience, and operational efficiency.
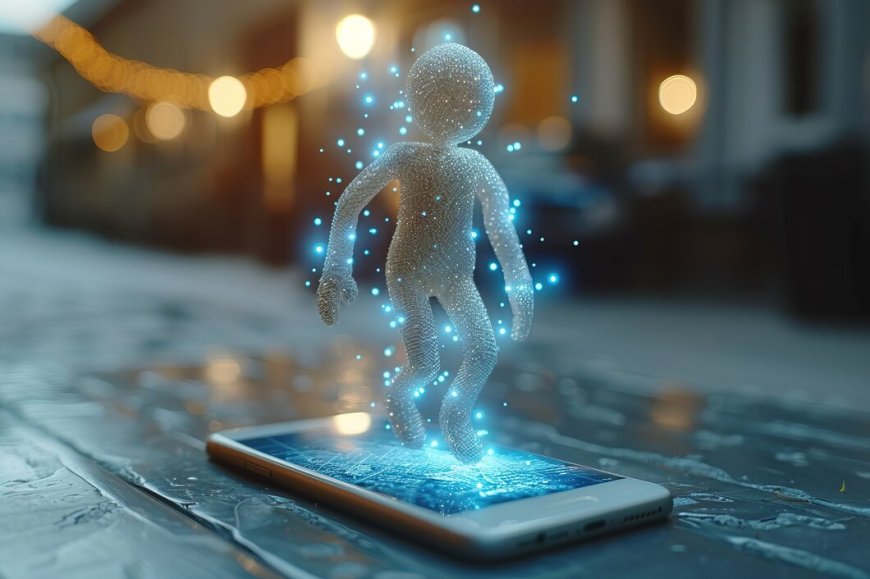
AI chatbots are transforming digital communication, providing instant responses and automating repetitive tasks. Their integration into messaging platforms like Telegram has seen a significant rise due to the platform's open API and extensive user base.
Automation and AI play a crucial role in modern communication by enhancing user interaction, improving response times, and managing vast amounts of data effectively. Python, MySQL, and the OpenAI API together form an excellent technology stack for developing a Telegram chatbot, with Python’s simplicity, MySQL’s efficient data management capabilities, and OpenAI’s advanced language model.
What is a Telegram Chatbot? A Telegram chatbot is a software program designed to automate conversations and perform tasks within the Telegram messaging app. These bots can be used for customer support, information delivery, and task automation.
Telegram provides the Telegram Bot API, which enables developers to build and manage chatbots efficiently. This API serves as the primary interface for sending and receiving messages from the bot users.
Benefits of Developing an AI Chatbot for Telegram:
-
Automation: Reduces manual efforts and streamlines repetitive tasks.
-
Enhanced User Experience: Provides quick responses and 24/7 availability.
-
Operational Efficiency: Handles multiple users simultaneously without human intervention.
Tools and Technologies Required: To build an AI-powered Telegram chatbot, the following tools are essential:
-
Python: A versatile programming language for scripting and automation.
-
MySQL: A relational database for data storage and management.
-
Telegram Bot API: Core API for bot interaction with Telegram users.
-
OpenAI API: For advanced language understanding and natural language generation.
Step-by-Step Development Process:
-
Setting up a Telegram Bot via BotFather:
-
Open the Telegram app and search for "BotFather."
-
Start a chat with BotFather and use the command
/newbot
to create a new bot. -
Follow the instructions and note down the API token provided.
-
-
Configuring Python Environment:
-
Download and install Python from the official website.
-
Set up a virtual environment using
python -m venv venv
.
-
-
Installing Required Libraries:
-
Activate your virtual environment with
source venv/bin/activate
(Linux/macOS) orvenv\Scripts\activate
(Windows). -
Install necessary libraries using the command:
pip install python-telegram-bot mysql-connector-python openai
.
-
-
Integrating Python with MySQL Database:
-
Create a MySQL database using tools like phpMyAdmin or MySQL Workbench.
-
Create a table to store chatbot responses:
-
CREATE TABLE responses (
id INT PRIMARY KEY AUTO_INCREMENT,
keyword VARCHAR(100),
response_text VARCHAR(255)
);
-
Connect to the database from Python:
import mysql.connector
conn = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='chatbot_db'
)
cursor = conn.cursor()
-
Integrating OpenAI API for Intelligent Responses:
-
Get your OpenAI API key from OpenAI.
-
Use the OpenAI API for generating responses:
-
import openai
openai.api_key = 'your_openai_api_key'
def get_openai_response(prompt):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=150
)
return response.choices[0].text.strip()
-
Implementing Basic Chatbot Functionalities:
-
Create a basic bot script using the Telegram Bot API:
-
from telegram import Update
from telegram.ext import Updater, CommandHandler, CallbackContext
def start(update: Update, context: CallbackContext):
update.message.reply_text('Hello! I am your chatbot.')
updater = Updater('YOUR_TELEGRAM_API_TOKEN')
updater.dispatcher.add_handler(CommandHandler('start', start))
updater.start_polling()
updater.idle()
-
Enhancing Chatbot with NLP Features via OpenAI API:
-
Enhance the bot to use OpenAI responses:
-
from telegram.ext import MessageHandler, Filters
def handle_message(update: Update, context: CallbackContext):
user_message = update.message.text
ai_response = get_openai_response(user_message)
update.message.reply_text(ai_response)
updater.dispatcher.add_handler(MessageHandler(Filters.text & ~Filters.command, handle_message))
Python and MySQL Integration: Connecting Python to MySQL for efficient data storage and retrieval is straightforward. Here’s a basic example:
import mysql.connector
db = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="chatbot_db"
)
cursor = db.cursor()
cursor.execute("SELECT * FROM responses")
for row in cursor.fetchall():
print(row)
Deploying the AI-Powered Chatbot: To keep the chatbot running continuously:
-
Use cloud hosting platforms like Heroku, AWS, or PythonAnywhere.
-
Implement Python’s
schedule
andtime
libraries for automation. -
Ensure the bot restarts automatically using tools like PM2 or systemd.
Use Cases and Real-World Applications:
-
Customer Support: Automated responses for common queries.
-
E-commerce Automation: Order tracking, product recommendations.
-
Content Delivery and Notifications: News updates, event reminders.
Conclusion: Developing an AI chatbot for Telegram using Python, MySQL, and OpenAI API offers numerous benefits, including automation, improved efficiency, and enhanced user experiences. By following the outlined steps, developers can build powerful chatbots capable of handling various tasks.
Explore Python scripting, database management, and advanced AI technologies further to enhance your chatbot development skills.