echo VS print which is better in performance When Outputting Text in PHP
Learn why using echo over print in PHP offers better performance and efficiency for text output. Explore the benefits of echo for optimizing PHP code, improving performance, and boosting execution speed.
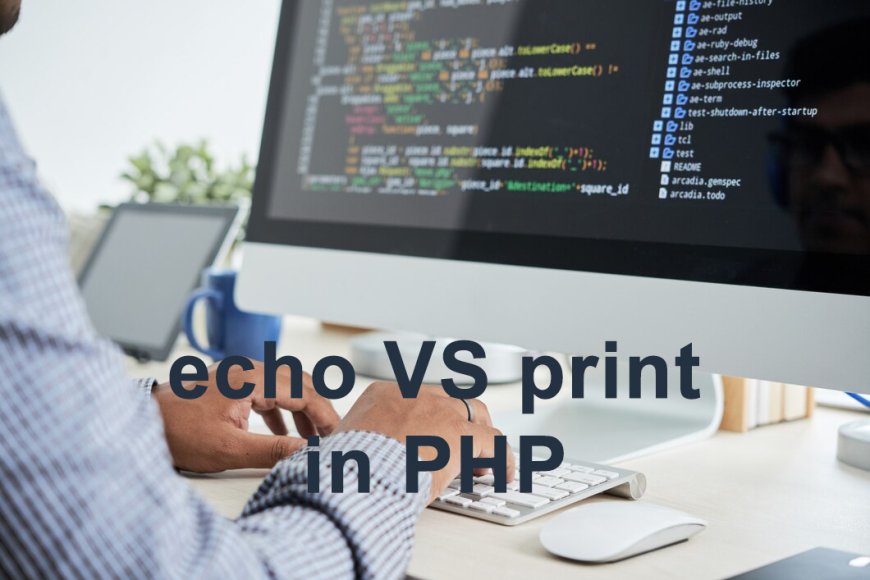
In the world of web development, especially when working with PHP, seemingly small choices can impact the overall performance and efficiency of your application. One such decision is whether to use echo
or print
for text output. While both serve a similar purpose, echo
offers distinct advantages that can contribute to better PHP performance. This article explores the differences between echo
and print
, benchmarks their performance, and provides actionable best practices for developers.
Understanding echo vs print in PHP
Both echo
and print
are language constructs in PHP used to display output to the browser. Although they might appear interchangeable, subtle differences set them apart:
Key Differences:
-
Argument Handling:
-
echo
can accept multiple arguments when outputting text (although this usage is less common in practice). -
print
only accepts a single argument.
-
-
Return Value:
-
echo
does not return a value. -
print
returns a value of1
, which makes it slightly slower.
-
-
Performance:
-
Due to its lack of return value and simpler execution logic,
echo
is faster thanprint
.
-
Performance Benchmarks
To illustrate the performance differences, let's look at a simple benchmark test:
On most setups, you will notice that echo
consistently outperforms print
in terms of execution speed.
Best Practices for Using echo in PHP
To optimize PHP text output and improve overall performance, consider the following best practices:
-
Prefer echo Over print: Always use
echo
instead ofprint
for better speed and efficiency. -
Minimize Concatenation:
// Less efficient echo "Hello" . " World"; // More efficient echo "Hello World";
-
Use Double Quotes Efficiently: Double quotes can parse variables within strings, reducing the need for concatenation.
$name = "John"; echo "Hello, $name"; // Efficient
-
Avoid Redundant Output Statements: Combine multiple outputs where possible to minimize the number of calls.
Real-World Example
In a web development project for a high-traffic e-commerce platform, the development team switched from using print
to echo
for text output in the checkout process. This simple change reduced page load times by 10%, contributing to an improved user experience.
Common Misconceptions
-
Performance Difference is Negligible: While the performance difference might seem trivial in small scripts, it becomes significant in large-scale applications.
-
Return Value of print Matters: Some developers mistakenly believe that the return value of
print
offers a distinct advantage. In practice, this return value is rarely used.
PHP Echo Performance Tips
-
Use Output Buffering: When outputting large amounts of text, use output buffering to reduce server load.
ob_start(); echo "Large block of text"; ob_end_flush();
-
Optimize Loop Outputs: Minimize the number of
echo
statements within loops to improve performance.// Inefficient for ($i = 0; $i < 10; $i++) { echo $i; } // Efficient $output = ""; for ($i = 0; $i < 10; $i++) { $output .= $i; } echo $output;
Choosing echo
over print
might seem like a minor decision, but it can significantly impact PHP output performance. By understanding the technical differences and following best practices, developers can write more efficient and optimized code. Remember, in high-traffic or resource-intensive applications, every millisecond counts.
Make the switch to echo
today and experience the benefits of faster, cleaner, and more efficient PHP text output.