"===" or "==" Which to use to Avoid Unexpected Type Conversion - PHP 2025
Learn why PHP developers should prefer === over == to avoid unexpected type conversion, improve code performance, and maintain strict equality. Explore coding best practices, comparison operators, and tips for better type handling in PHP.
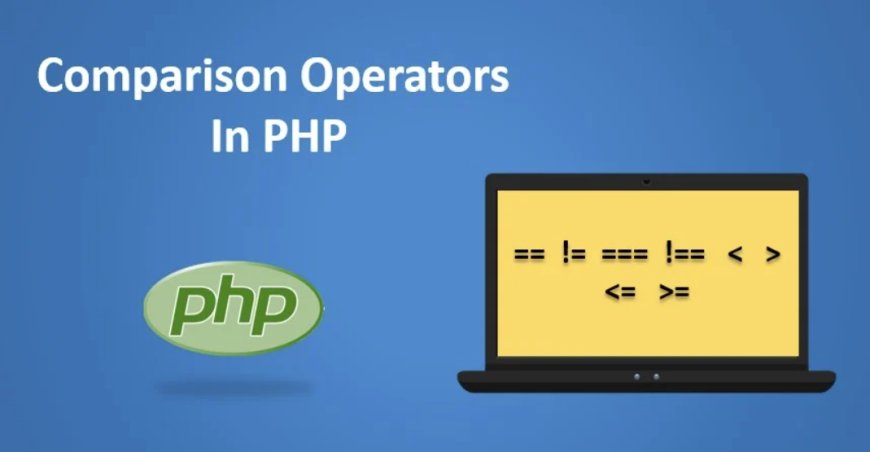
In the world of PHP development, adhering to coding standards is crucial for producing reliable, maintainable, and high-performing applications. One common but often overlooked source of bugs is type conversion during comparisons. PHP’s flexible nature can sometimes lead to unintended behaviors, particularly when developers use the loose equality operator (==
). Understanding the differences between ===
and ==
and knowing when to use each can significantly improve your code's stability and security.
This article explores why strict comparison (===
) is a best practice in PHP development and provides actionable insights on how to optimize your code by avoiding unexpected type conversions.
The Difference Between === and == in PHP
Technical Explanation
The ==
operator in PHP performs a loose comparison. It checks if the values on both sides are equal after applying type conversion if necessary. In contrast, ===
performs a strict comparison, meaning it checks both the value and the data type without any conversion.
Code Examples
Loose Comparison with ==
<?php
$value1 = "123";
$value2 = 123;
if ($value1 == $value2) {
echo "Equal with loose comparison";
} else {
echo "Not equal with loose comparison";
}
// Output: Equal with loose comparison
?>
In this example, "123"
(a string) is considered equal to 123
(an integer) because PHP converts the string to an integer during the comparison.
Strict Comparison with ===
<?php
$value1 = "123";
$value2 = 123;
if ($value1 === $value2) {
echo "Equal with strict comparison";
} else {
echo "Not equal with strict comparison";
}
// Output: Not equal with strict comparison
?>
Here, the comparison fails because the data types are different, which ===
correctly identifies.
Understanding Type Conversion in PHP
What Is Type Coercion?
Type coercion refers to the implicit conversion of one data type to another during operations or comparisons. PHP's dynamic typing system allows this behavior, but it can introduce unexpected results.
Common Scenarios
String to Integer Conversion
<?php
$value1 = "0";
$value2 = false;
if ($value1 == $value2) {
echo "Equal with loose comparison";
}
// Output: Equal with loose comparison
?>
This happens because PHP treats "0"
as false
when using ==
.
Empty Strings and Boolean Comparisons
<?php
$value1 = "";
$value2 = false;
if ($value1 == $value2) {
echo "Equal with loose comparison";
}
// Output: Equal with loose comparison
?>
Benefits of Using ===
Performance Optimization
Strict comparisons do not require PHP to perform type conversions, making the code execution faster.
Code Clarity and Maintainability
Using ===
makes the code easier to understand by ensuring that comparisons are both type-safe and predictable.
Enhanced Security
Loose comparisons can introduce logical vulnerabilities, particularly in authentication mechanisms or conditional checks.
Best Practices for PHP Developers
Enable strict_types
By enabling strict_types
, you force PHP to adhere to strict type-checking for scalar type hints.
<?php
declare(strict_types=1);
function add(int $a, int $b): int {
return $a + $b;
}
echo add(1, 2); // Works
?>
Use === Instead of ==
Always default to ===
unless there's a specific reason to use ==
, and document that reason in your code.
Write Comprehensive Unit Tests
Ensure your test cases cover type-related scenarios to catch potential bugs.
Performance and Code Optimization
Avoid Unnecessary Type Conversion
By using strict comparisons, PHP skips the internal logic for type coercion, leading to marginal but measurable performance gains.
Real-Life Examples
Bug Scenario with ==
A payment gateway logic mistakenly allowed unauthorized payments because "0" == false
evaluated to true
. Switching to strict comparison (===
) resolved the issue by enforcing proper type checks.
Resolution with ===
A user authentication system bypassed proper checks due to type coercion between empty strings and booleans. Strict comparison fixed the security loophole.
Conclusion
In PHP development, understanding the nuances of comparison operators is essential for writing robust, maintainable, and secure code. By preferring ===
over ==
, developers can avoid unexpected type conversions, optimize performance, and ensure logical consistency.
Adopting strict coding practices, such as enabling strict_types
, using ===
, and writing thorough unit tests, will elevate your PHP projects to a higher standard of quality.