PHP Performance Optimization Techniques: Custom PHP Development
Discover essential PHP performance optimization techniques for custom PHP development. Learn how to optimize PHP code, reduce load times, and improve website speed with expert PHP development tips and best practices.
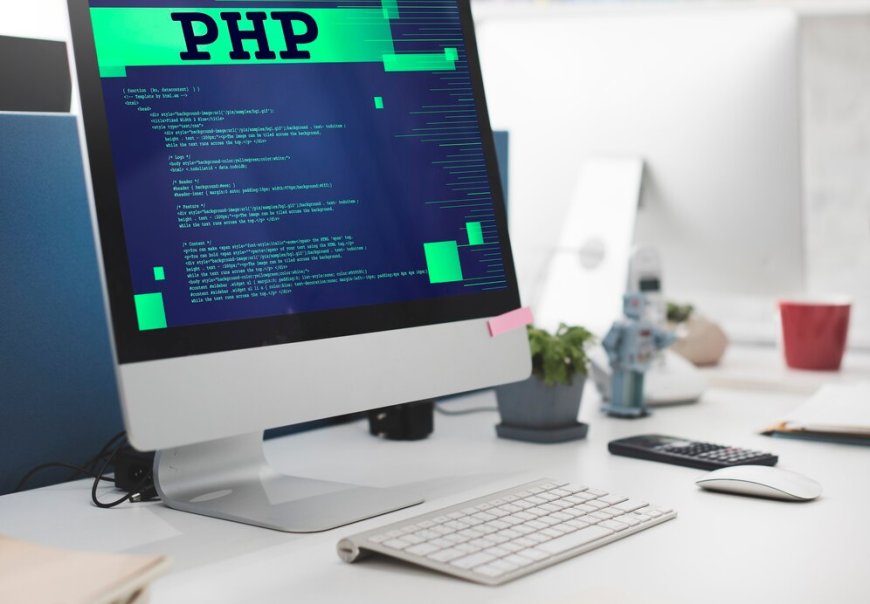
PHP performance optimization is essential for ensuring fast load times, better user experience, and efficient server performance. Custom PHP development provides flexibility, but poorly optimized code can slow down websites and affect business outcomes.
This guide explores proven PHP performance optimization techniques with step-by-step instructions to improve your PHP website's speed and efficiency.
Why PHP Performance Optimization Matters
- User Experience: Faster websites keep users engaged.
- SEO Rankings: Google rewards fast-loading websites.
- Server Efficiency: Optimized code uses fewer resources.
- Business Impact: Faster websites boost conversions and customer retention.
Key PHP Performance Optimization Techniques (Step-by-Step)
1. Optimize PHP Code
Steps to Optimize PHP Code:
-
Minimize Redundancy:
- Avoid repeated database queries inside loops.
- Store results in variables instead.
-
Use Object-Oriented Programming (OOP):
- Break down code into reusable classes and methods.
- Example:
-
Remove Unnecessary Functions:
- Avoid functions doing the same task multiple times.
2. Implement PHP Caching Techniques
Caching reduces server load by storing processed data for reuse.
Steps to Enable OPcache (Opcode Caching):
- Open your php.ini file.
- Enable OPcache by adding:
- Restart your web server:
Steps to Enable Data Caching with Redis:
- Install Redis on your server:
- Connect Redis in PHP:
3. Use PHP Performance Tools for Debugging
Steps to Install and Use Xdebug for Profiling:
- Install Xdebug:
- Enable profiling in php.ini:
- Restart your server and use tools like Webgrind to visualize profiling results.
4. Optimize Database Queries
Steps to Optimize SQL Queries in PHP:
- Use Indexed Tables:
- Optimize Queries:
- Avoid SELECT * Queries:
- Fetch only required columns instead.
5. Reduce PHP Load Time with Minification
Steps to Minify CSS, JS, and HTML:
-
Use Online Tools: Use tools like Minify to compress code.
-
Enable GZIP Compression:
- Edit
.htaccess
:
- Edit
-
Use PHP Code Minifiers:
6. Enable Content Delivery Network (CDN)
Steps to Implement a CDN:
- Choose a CDN provider: Cloudflare, KeyCDN, or BunnyCDN.
- Integrate the CDN:
- Update DNS settings in your domain registrar.
- Serve Static Files via CDN:
- Modify resource links:
7. PHP Version Upgrade (Latest Stable Release)
Steps to Upgrade PHP:
- Check Current PHP Version:
- Upgrade PHP on Ubuntu (Example for PHP 8.2):
Best Practices for Custom PHP Development
- Use Frameworks: Prefer optimized frameworks like Laravel or Symfony.
- Follow Coding Standards: Adhere to PSR-12 standards.
- Minimize External Libraries: Use only necessary plugins and libraries.
- Optimize File Structure: Organize code into modules for better readability and performance.
PHP Performance Checklist for Developers
✔️ Enable OPcache and Redis caching.
✔️ Optimize SQL queries and avoid SELECT *
.
✔️ Minify CSS, JS, and HTML files.
✔️ Use Xdebug for performance profiling.
✔️ Use the latest PHP version for better performance.
✔️ Enable GZIP compression.
✔️ Use CDN for faster content delivery.
PHP performance optimization is crucial for delivering fast, scalable, and secure web applications. By following these step-by-step optimization techniques, you can improve website speed, enhance user experience, and reduce server load.
Start implementing these strategies today to make your PHP websites faster and more efficient! ????
Need help with PHP optimization? Visit https://www.intelliness.in/ and hire a professional PHP developer for custom solutions tailored to your business needs.