PHP Tags: What to use for compatibility across servers
Learn the key differences between PHP full tags and short tags, and discover best practices for ensuring PHP compatibility across servers. Find out which tag is recommended for optimized, cross-server web development and adherence to coding standards.
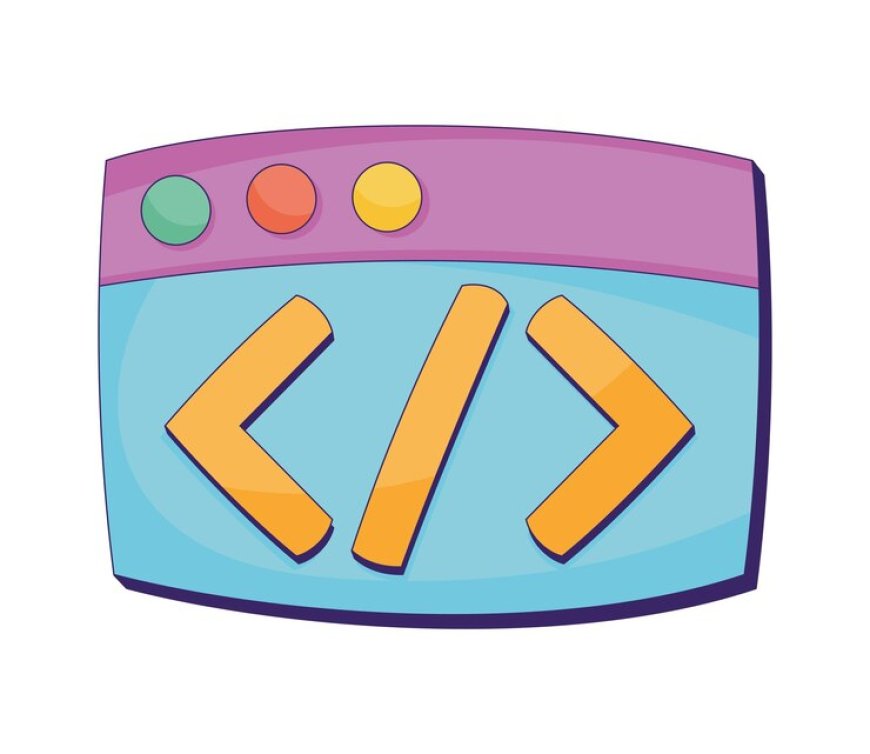
PHP (Hypertext Preprocessor) is one of the most widely used server-side scripting languages in web development. Its versatility, ease of use, and powerful features make it a go-to choice for developers around the world. However, one crucial aspect of writing maintainable and compatible PHP code often goes overlooked—PHP tag usage. Selecting the right PHP tags can significantly impact your code's compatibility across different server environments.
In this article, we will explore the differences between full and short PHP tags, their compatibility considerations, best practices for tag usage, and tips for ensuring smooth PHP development across various server configurations.
Types of PHP Tags
PHP offers several ways to open and close code blocks. Understanding these options is essential for writing flexible and compatible scripts.
1. Full PHP Tags (<?php ?>
)
This is the most standard and widely supported way to open and close PHP code.
<?php
echo "Hello, World!";
?>
2. Short PHP Tags (<? ?>
)
Short tags are a shorthand way to include PHP but are less commonly supported.
<?
echo "Hello, World!";
?>
3. Short Echo Tags (<?= ?>
)
These tags are a convenient shortcut for <?php echo ?>
.
<?= "Hello, World!" ?>
Note: Short echo tags are always enabled starting from PHP 5.4.
Compatibility and Server Considerations
1. Server Configurations and Compatibility Issues
One of the primary concerns with PHP short tags (<? ?>
) is their dependency on server configuration. The short_open_tag
directive in the php.ini
file controls whether short tags are supported. By default, many servers disable this directive, especially in shared hosting environments.
If a server does not support short tags and the directive is disabled, your PHP code will not execute as expected, potentially leading to syntax errors or broken functionality.
2. PHP Version Requirements
-
Full PHP tags (
<?php ?>
) are universally supported across all PHP versions and server configurations. -
Short echo tags (
<?= ?>
) are safe to use since they are enabled by default from PHP 5.4 onwards. -
Avoid using short tags (
<? ?>
) to ensure broader compatibility.
Best Practices and Coding Standards
1. Use Full PHP Tags (<?php ?>
)
The PHP community and coding standards, including PSR-1 and PSR-12, recommend using full tags for maximum compatibility.
Example:
<?php
// This is a portable and maintainable PHP block
echo "Best practice example.";
?>
2. Consistent Tag Usage
Maintain consistent tag usage across your projects to enhance readability and maintainability.
3. Avoid Short Tags (<? ?>
)
Using short tags can lead to compatibility issues and is generally discouraged.
Performance and Code Optimization
While some developers argue that using short tags might marginally reduce file size, the impact on performance is negligible. PHP interpreters efficiently process both full and short tags without noticeable performance differences.
To optimize PHP code while maintaining compatibility:
-
Use caching mechanisms like OPcache.
-
Minimize unnecessary code execution.
-
Follow PHP coding best practices for maintainable and efficient scripts.
Real-World Development Tips
1. Configuring Servers for PHP Compatibility
If you have control over the server, you can enable short tags by updating the php.ini
file:
short_open_tag = On
However, relying on this configuration is risky, especially when deploying to shared hosting or client-controlled environments.
2. Development Environment Considerations
-
Set up development environments that mimic production servers.
-
Ensure configurations are consistent across development, testing, and production stages.
3. Deployment Strategies
-
Use automated deployment tools to verify server compatibility.
-
Include compatibility checks as part of your CI/CD pipeline.
Conclusion
In web development, ensuring PHP script compatibility across different servers is crucial for maintaining functional, reliable applications. Using full PHP tags (<?php ?>
) is the best practice for achieving broader compatibility, adhering to coding standards, and avoiding server configuration pitfalls.
Short echo tags (<?= ?>
) are generally safe, but it’s best to steer clear of short PHP tags (<? ?>
) to prevent potential compatibility issues.
By following these guidelines and development tips, you can write robust, maintainable PHP code that works seamlessly across various server environments, ensuring a smoother development experience and better user satisfaction.