Top PHP Built-in Functions for Memory Optimization
Explore the top PHP built-in functions that optimize memory usage, enhance performance, and improve scalability in resource-intensive applications. This guide offers practical tips, coding examples, and best practices for PHP developers to manage memory efficiently.
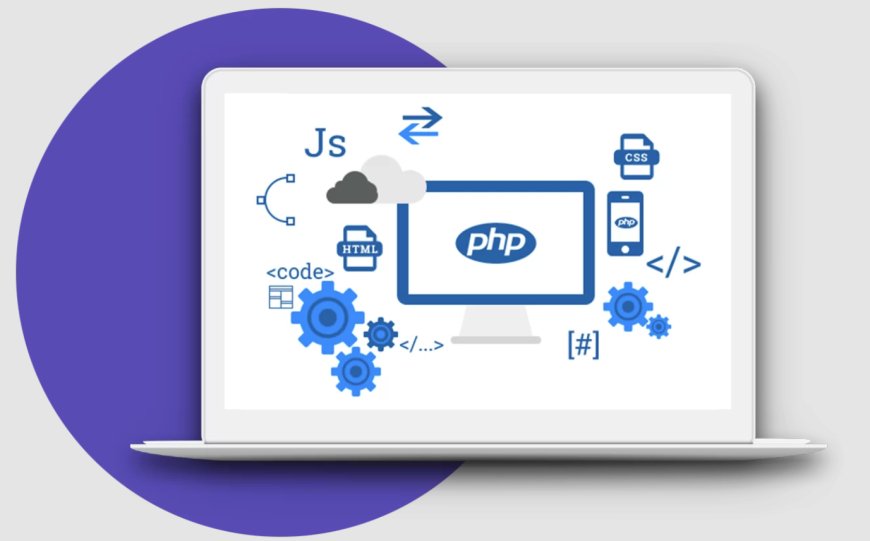
In the fast-paced world of web development, performance matters. Memory optimization is a critical aspect of PHP development, especially for resource-intensive applications that handle large datasets, complex calculations, or high traffic. By leveraging PHP’s built-in functions, developers can significantly enhance their application’s performance and scalability. In this article, we’ll explore the top PHP built-in functions for memory optimization and share practical tips for efficient memory management.
The Importance of Memory Optimization in PHP
Memory optimization ensures that your PHP application runs efficiently without consuming excessive server resources. Poor memory management can lead to slower performance, server crashes, or increased hosting costs. This is particularly crucial for:
-
High-traffic websites: Minimizing memory usage ensures smooth operation under heavy loads.
-
Data-intensive applications: Handling large datasets efficiently avoids bottlenecks.
-
Long-running scripts: Preventing memory leaks is essential for stable execution.
By understanding and utilizing PHP’s built-in functions, developers can monitor, manage, and optimize memory usage effectively.
Top PHP Built-in Functions for Memory Optimization
1. memory_get_usage()
-
Purpose: Measures the current memory usage of a script.
-
Use Case: Monitor memory consumption during debugging or optimization.
-
Example:
echo "Current memory usage: " . memory_get_usage() . " bytes";
2. memory_get_peak_usage()
-
Purpose: Tracks the peak memory usage during script execution.
-
Use Case: Identify high-memory operations for further optimization.
-
Example:
echo "Peak memory usage: " . memory_get_peak_usage() . " bytes";
3. gc_collect_cycles()
-
Purpose: Triggers PHP’s garbage collector to free unused memory.
-
Use Case: Manage memory in long-running scripts or applications.
-
Example:
if (gc_enabled()) { gc_collect_cycles(); }
4. unset()
-
Purpose: Frees up memory by unsetting variables.
-
Use Case: Remove unused variables, especially in loops or large datasets.
-
Example:
unset($largeArray);
5. serialize() and unserialize()
-
Purpose: Store and retrieve data efficiently with minimized memory usage.
-
Use Case: Cache data between script executions.
-
Example:
$serializedData = serialize($data); $unserializedData = unserialize($serializedData);
6. array_chunk()
-
Purpose: Splits large arrays into smaller, manageable chunks.
-
Use Case: Process large datasets in memory-efficient batches.
-
Example:
$chunks = array_chunk($largeArray, 100); foreach ($chunks as $chunk) { process($chunk); }
7. array_filter() and array_map()
-
Purpose: Apply functions directly to arrays without creating unnecessary copies.
-
Use Case: Manipulate data efficiently.
-
Example:
$filtered = array_filter($array, fn($value) => $value > 10); $mapped = array_map(fn($value) => $value * 2, $array);
8. fread()
-
Purpose: Reads files in chunks instead of loading entire files into memory.
-
Use Case: Work with large files like logs or data dumps.
-
Example:
$handle = fopen("largefile.txt", "r"); while (($line = fread($handle, 4096)) !== false) { process($line); } fclose($handle);
9. json_encode() and json_decode()
-
Purpose: Handle structured data efficiently with minimal memory overhead.
-
Use Case: Store and process lightweight data formats.
-
Example:
$jsonData = json_encode($data); $decodedData = json_decode($jsonData, true);
10. count() with Conditions
-
Purpose: Use
count($array, COUNT_RECURSIVE)
to avoid unnecessary iterations. -
Use Case: Optimize nested array processing.
-
Example:
$totalElements = count($nestedArray, COUNT_RECURSIVE);
Best Practices for PHP Memory Optimization
-
Use Efficient Algorithms: Optimize logic to reduce complexity.
-
Optimize Database Queries: Fetch only the necessary data.
-
Implement Lazy Loading: Load resources only when needed.
-
Use Pagination: Break down large datasets into manageable pages.
-
Leverage Caching: Use tools like OPcache or Memcached for faster data access.
-
Monitor Memory Usage: Continuously track memory consumption during development and testing.
Common Pitfalls to Avoid
-
Excessive Variables: Avoid loading unnecessary data into memory.
-
Memory Leaks: Regularly test for leaks, especially in long-running applications.
-
Large Datasets: Process data in smaller chunks to prevent memory exhaustion.
Optimizing memory usage in PHP applications is crucial for creating high-performance, scalable solutions. By leveraging PHP’s built-in functions and following best practices, developers can significantly reduce memory consumption, enhance application speed, and improve user experiences.